April 2022 Newsletter
[TODO some description]
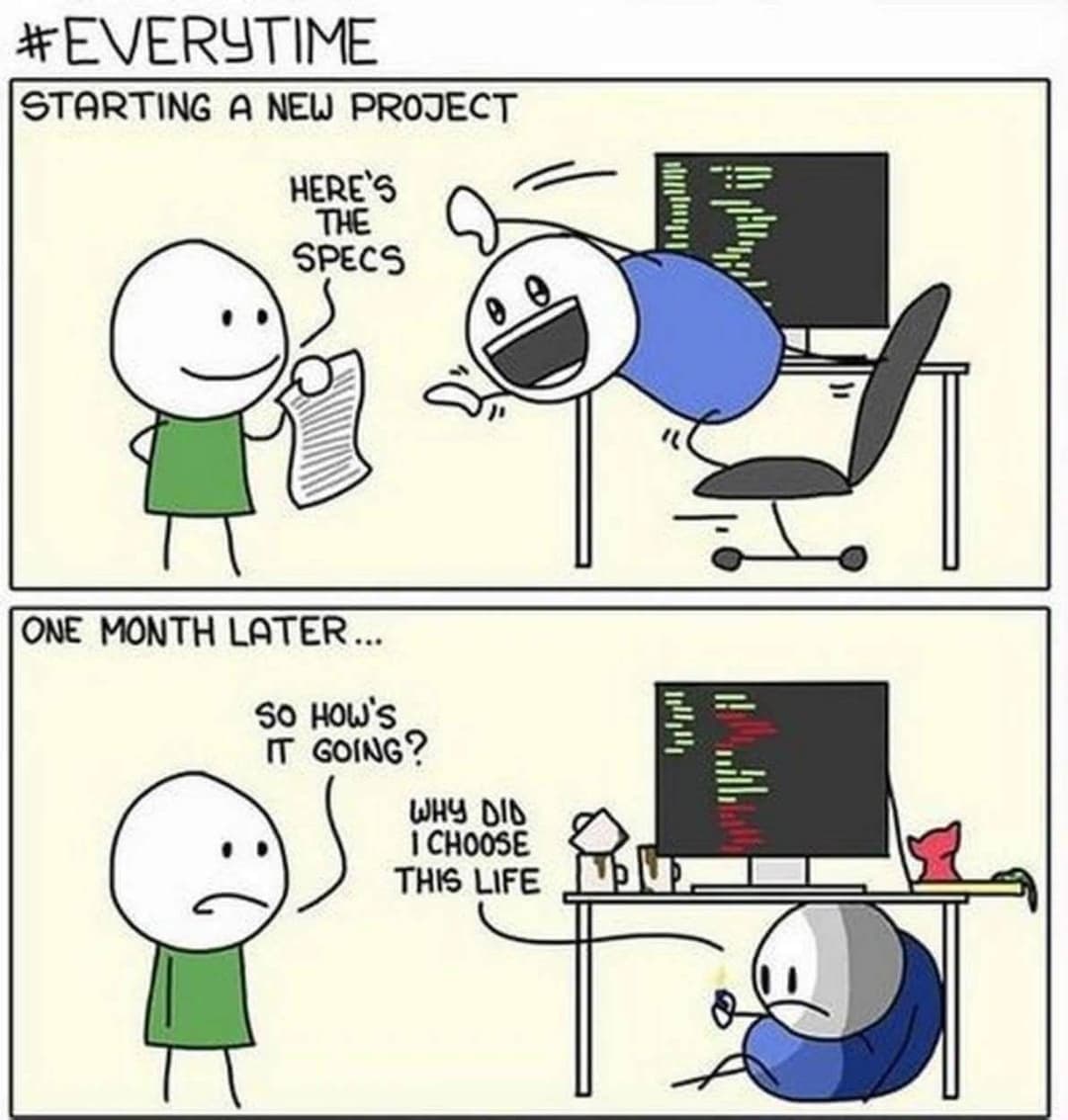
Contents š
News & Explore
As new projects are kicking off and ongoing projects are scaling up, different problems have arisen. The ecosystem is growing a lot with new versions and updates. Letās see what we have this month.
Flexible components using as/is
While using styled-components, we are using āasā prop to change the HTML element that is rendered. Ever wonder how you can do that?
Flexible Design Systems (opens in a new tab)
Node 18 has now released
Node.js is bringing bigger features, updates, and fixes. Some of the features that are coming:
- global fetch(experimental)
- runner module for testing
Text Editor from Facebook
When users need to put text with edits and formatting, itās one of the things that is problematic. Facebook released a text editor framework that does things differently.
GitHub Repo (opens in a new tab)
JavaScript operatorsā¦
If you want to quickly name 5 operators, how many can you name? There are 50 operators in JS. If you are surprised as I am, let me show you all.
Operator Lookup (opens in a new tab)
Fetch or Axios
The battle of request libraries is on. Which one do you think will win?
Fetch vs Axios (opens in a new tab)
Throwing everything in JS
Did you know you can literally throw everything in javascript?
Throw Anything (opens in a new tab)
Rome formatter
Over the last year, Rome has been rewritten into rust and it seems like it will overrule Eslint and others for formatting.
Announcing Rome Formatter (opens in a new tab)
New Array methods
Creating array from an array, using references, making changes on copied ones⦠Those long processes might be replaced.
Hackernoon Post (opens in a new tab)
Need a blog but donāt have time?
I've got your back. Hereās a ready-to-use blog template for you built with Tailwind and Next.js.
GitHub Repo (opens in a new tab)
Redwood.js 1.0 is out
Redwood is a full-stack React framework for your needs, and version 1 is officially out.
RedwoodJS v1.0 Launch (opens in a new tab)
AI creating images from text?
Should we be alarmed or just be fine with it?
DALLĀ·E 2 (opens in a new tab)
Terms of service; Didnāt read
Itās hard to read all of the TOS on each application, so thereās an easier way.
Frontpage -- Terms of Service; Didn't Read (opens in a new tab)
Libraries
Prisma: has released v3.13.0 (opens in a new tab)
migrate diff and db execute are now Generally Available!
Remotion: has released v3.0 (opens in a new tab)
After more than 10 months in development and 1400 commits, remotion has been reworked.
Jest: has released v28 (opens in a new tab)
Fake timers are default, Github Actions reporter, sharding on tests.
Ember: has released v4.3 (opens in a new tab)
No features and no deprecations, just some bug fixes.
Nuxt: has released v3-RC (opens in a new tab)
The first release candidate of version 3 has been announced.
Challenge
We are not using Redux every day, but still, the reducer pattern is something we can use in React directly. And they might have some problems. So letās see if you can figure that out. (If you run code it will tell you immediately actually š )
function todoReducer(state, action) {
switch (action.type) {
case "ADD_TODO":
const todos = state.todos;
return { ...state, todos: todos.concat(action.payload) };
case "REMOVE_TODO":
const todos = state.todos;
const newTodos = todos.filter((todo) => todo.id !== action.id);
return { ...state, todos: newTodos };
default:
return state;
}
}
Explanation
const
and let
are block-scoped. It means they need to have different scope for the same variable name for todos , or you can change the name of the variable. The new scope is defined with after ācase".
function todoReducer(state, action) {
switch (action.type) {
case "ADD_TODO": {
const todos = state.todos;
return { ...state, todos: todos.concat(action.payload) };
}
case "REMOVE_TODO": {
const todos = state.todos;
const newTodos = todos.filter((todo) => todo.id !== action.id);
return { ...state, todos: newTodos };
}
default:
return state;
}
}